Handling promises
toast.promiseβ
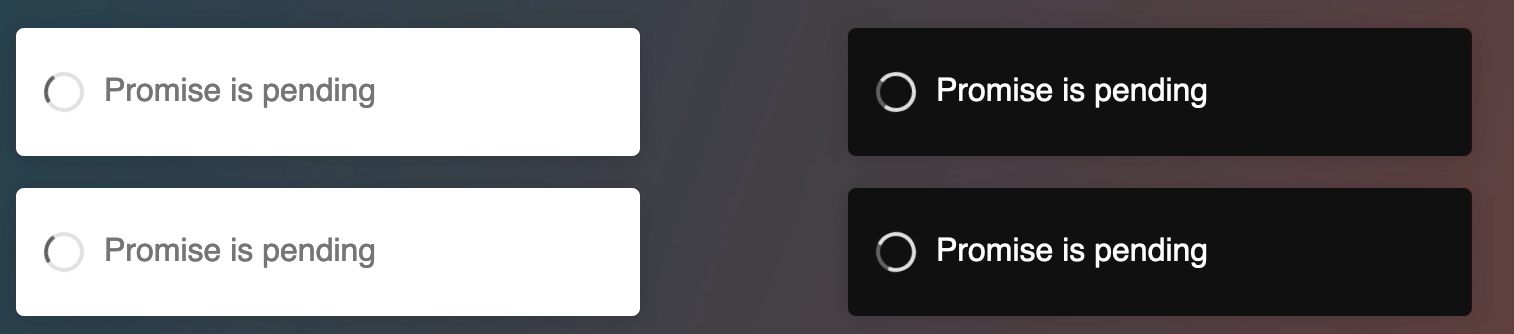
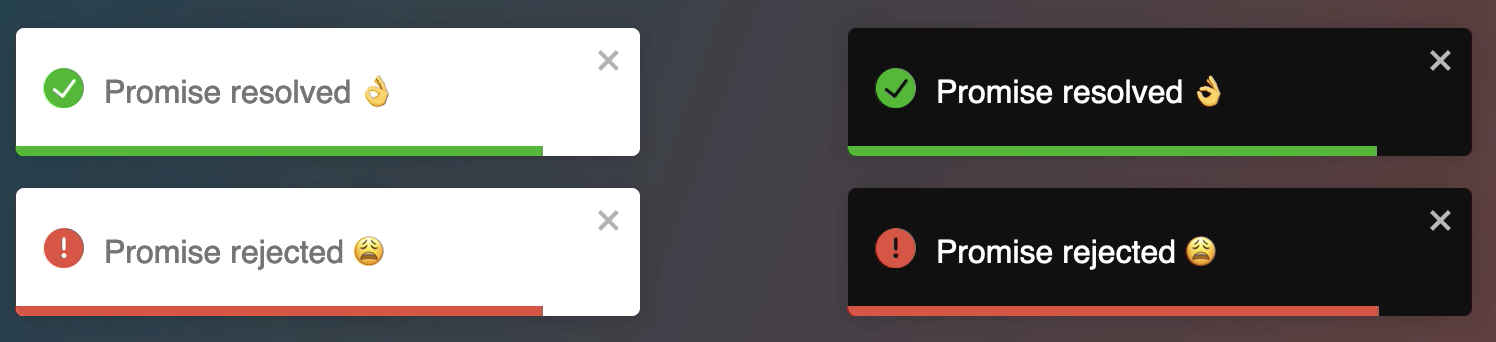
The library exposes a toast.promise
function. Supply a promise or a function that return a promise and the notification will be updated if it resolves or fails. When the promise is pending a spinner is displayed.
Let's start with a simple example
const resolveAfter3Sec = new Promise(resolve => setTimeout(resolve, 3000));
toast.promise(
resolveAfter3Sec,
{
pending: 'Promise is pending',
success: 'Promise resolved π',
error: 'Promise rejected π€―'
}
)
const functionThatReturnPromise = () => new Promise(resolve => setTimeout(resolve, 3000));
toast.promise(
functionThatReturnPromise,
{
pending: 'Promise is pending',
success: 'Promise resolved π',
error: 'Promise rejected π€―'
}
)
toast.promise return the provided promise so you can chain it
const response = await toast.promise(
fetch("A_URL"),
{
pending: 'Promise is pending',
success: 'Promise resolved π',
error: 'Promise rejected π€―'
}
);
console.log(response)
Displaying a simple message is what you would want to do in 90% of cases. But what if the message you want to display depends on the promise response, what if you want to change some options for the error notification? Rest assured, under the hood, the library uses toast.update
. Thanks to this, you have full control over each notification.
const resolveWithSomeData = new Promise(resolve => setTimeout(() => resolve("world"), 3000));
toast.promise(
resolveWithSomeData,
{
pending: {
render(){
return "I'm loading"
},
icon: false,
},
success: {
render({data}){
return `Hello ${data}`
},
// other options
icon: "π’",
},
error: {
render({data}){
// When the promise reject, data will contains the error
return <MyErrorComponent message={data.message} />
}
}
}
)
toast.update
, you can even supply a custom transition if you want π€―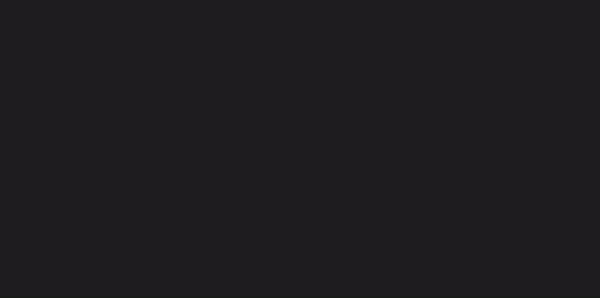
toast.loadingβ
If you want to take care of each step yourself you can use toast.loading
and update the notification yourself.
const id = toast.loading("Please wait...")
//do something else
toast.update(id, { render: "All is good", type: "success", isLoading: false });